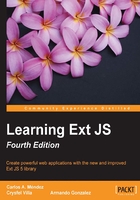
Working with the DOM
Ext JS provides an easy way to deal with the DOM. We can create nodes, change styles, add listeners, and create beautiful animations, among other things without worrying about the browser's implementations. Ext JS provides us with a cross-browser compatibility API that will make our lives easier.
The responsible class for dealing with the DOM nodes is the Ext.Element
class. This class is a wrapper for the native nodes and provides us with many methods and utilities to manipulate the nodes.
Note
Manipulating DOM directly is considered bad practice and none of the DOM markup should be placed in the index file. This example exists only for illustrative purposes.
Getting elements
The Ext.get
method let us retrieve a DOM element encapsulated in the Ext.dom.Element
class, retrieving this element by its ID. This will let us modify and manipulate the DOM element. Here is a basic example:
<!doctype html> <html> <head> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta charset="utf-8"> <title>Extjs - Loader</title> <link rel="stylesheet" type="text/css" href="../ext-5.1.1/build/packages/ext-theme-neptune/build/resources/ext-theme-neptune-all.css"> <script src="../ext-5.1.1/ext-all.js"></script> <script src="../ext-5.1.1/build/packages/ext-theme-neptune/build/ext-theme-neptune.js"></script> <script type="text/javascript"> Ext.onReady(function(){ var mymainDiv = Ext.get('main'); var mysecondDiv = Ext.dom.Element.get('second'); }); </script> </head> <body style="padding:10px"> <p id="main"></p> <p id="second"></p> </body> </html>
Usually to get an element, we use Ext.get
, which is an alias/shorthand for Ext.dom.Element.get
.
Note
When passing an ID, it should not include the # character that is used for a CSS selector.
In the p
variable, we have an instance of the Ext.Element
class containing a reference to the node that has main
as its ID.
We may use the setStyle
method in order to assign some CSS rules to the node. Let's add the following code to our example:
p.setStyle({ width: "100px", height: "100px", border: "2px solid #444", margin: "80px auto", backgroundColor: "#ccc" });
Here we are passing an object with all the rules that we want to apply to the node. As a result, we should see a gray square in the center of our screen:
If we want to add a CSS class to the node, we can use the addCls
method. We can also use the removeCls
method if we want to remove a CSS class from the node. Let's see how to use the addCls
method:
p.addCls("x-testing x-box-component"); p.removeCls("x-testing");
There are a lot of methods we can use to manipulate the node element. Let's try some animations with our element:
p.fadeOut() .fadeIn({ duration:3000 });
The fadeOut
method slowly hides the element by changing the opacity progressively. When the opacity is zero percent, the fadeIn
method is executed by changing the opacity by 100 percent in three seconds.
You should take a look at the documentation (http://docs.sencha.com/) in order to know all the options we have available, as there we can find examples of code to play with.
Query – how do we find them?
Ext JS allows us to query the DOM to search for specific nodes. The query engine supports most of the CSS3 selector specifications and the basic XPath.
The responsible class that does the job is the Ext.dom.Query
class; this class contains some methods to perform a search.
Note
The Ext.dom.Query
class is a singleton class so there is no need to declare it as a new instance to search DOM elements. Also it's important to know about CSS selectors, so this will help us to understand how we may select one or many elements.
The following code is an HTML document that contains a few tags so we can search for them using the Ext.dom.Query
class:
<!doctype html> <html> <head> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta charset="utf-8"> <title>Extjs - manipulating the DOM </title> <script src="../ext-5.1.1/build/ext-all.js"></script> <script type="text/javascript"> Ext.onReady(function(){ var myElements = Ext.dom.Query.select('#main .menu ul li'); myElements = Ext.get(myElements); myElements.setStyle({ display: "inline", backgroundColor: "#003366", margin: "3px", color: "#FFCC00", padding: "3px 20px", borderRadius: "10px", boxShadow: "inset 0 1px 15px #6699CC" }); var h1 = Ext.select("#main p[class=content] h1"); h1.setStyle("color","#003399"); }); </script> </head> <body style="padding:10px;"> <p id="main"> <p class="menu"> <ul> <li>Home</li> <li>About us</li> </ul> </p> <p class="content"> <h1>Learning Ext JS 5!</h1> <p>This is an example for the DomQuery class.</p> </p> </p> </body> </html>
In order to perform the search, we'll use the select
method from the Ext.dom.Query
class, and we pass a CSS selector as the only parameter, #main .menu ul li
. The myElements
variable became an array with two elements. Ext
wraps the nodes into an Ext.CompositeElementLite
collection.
After that, we convert the collection (each element in the array) to a Ext.dom.Element
object using the myElements = Ext.get(myElements);
instruction.
The myElements.setStyle({...});
instruction takes the action of applying the style (configuration object) to each one of the elements (in the array), using the Ext.dom.Element
methods to accomplish this. The following screenshot represents the result of the code:
DOM manipulation – how do we change it?
We can create and remove nodes from the DOM very easily. Ext JS contains a DomHelper
object/class, which provides an abstraction layer and gives us an API to create DOM nodes or HTML fragments.
Let's create an HTML file, import the Ext
library, and then use the DomHelper
object to append a p
element to the document's body:
Ext.onReady(function(){ Ext.DomHelper.append(Ext.getBody(),{ tag: "p", style: { width: "100px", height: "100px", border: "2px solid #333", margin : "20px auto" } }); });
We used the append
method; the first parameter is where we want to append the new element (or DOM node). In this case, we're going to append it to the document's body.
The second/next parameter is a string or object specifying the element that we are going to append; it's important that we specify the tag
property, which defines the type/kind of element (DOM element) that we desire to append to the element defined in the first parameter.
In this case, we previously defined a p
element to be appended in the document's body, but we can define any other tags as defined in the HTML specification. We can define styles, classes, children, and any other property that an HTML element supports. Let's add some children to our previous example:
Ext.DomHelper.append(Ext.getBody(),{ //... children : [{ tag : "ul", children : [ {tag: "li", html: "Item 1"}, {tag: "li", html: "Item 2"} ] }] });
We have added an unordered list to the main p
element. The list contains two children that are list elements. We can have as many children as needed.
There's another method that we can use if we want to create a node, but we want to insert it into the DOM later:
var h1 = Ext.DomHelper.createDom({ tag: "h1", html: "This is the title!" }); Ext.getBody().appendChild(h1);
When we use the createDom
method, we create a new node in the memory. We probably append this node to the DOM later on, or maybe not. In this example, we have appended it to the document's body.
We know how to create and append nodes to the DOM, but what if we want to remove elements from the DOM? In order to remove the element from the DOM, we need to use the remove
method on the Ext.Element
class:
Ext.fly(h1).remove();
The previous code is calling the Ext.fly
method. This method is similar to the Ext.get
method but the difference is that Ext.fly
gets the element and does not store this element in memory; really it's for a single use or a one time-reference. The Ext.get
method stores the element in memory to be reused in other classes or application code.
So Ext.fly
returns an instance to the Ext.Element
class containing a reference to the node element. Once we have the node in the wrapper, we can call the remove
method and the node will be removed from the DOM.