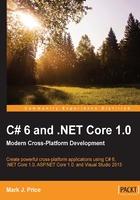
Understanding C# basics
Let's start with looking at the basics of the grammar and vocabulary of C#. In this chapter, you will create multiple console applications, each showing a feature of the C# language. To manage these projects, we will put them all in a single solution. Visual Studio 2015 can only have one solution open at any one time, but each solution can group together multiple projects. A project can build a console application, a Windows desktop application, a web application, and dozens of others.
Start Microsoft Visual Studio 2015. In Visual Studio, press Ctrl + Shift + N or choose the File | New | Project… menu.
In the New Project dialog, in the Installed Templates list, expand Other Project Types, and select Visual Studio Solutions. In the list at the center, select Blank Solution, type the name Chapter02, change the location to C:\Code
, and then click on OK, as shown in the following screenshot:

If you were to run File Explorer, you would see that Visual Studio has created a folder named Chapter02 with a Visual Studio solution named Chapter02 inside it, as follows:

In Visual Studio, navigate to File | Add | New Project…, as shown in the following screenshot. This will add a new project to the blank solution:

In the Add New Project dialog, in the Installed Templates list, select Visual C#. In the list at the center, select Console Application, type the name Ch02_Basics, ensure that .NET Framework 4.6 (or later) is selected at the top, and then click on OK.

If you were to run File Explorer, you would see that Visual Studio has created a new folder with some files and subfolders inside it. You don't need to know what all these do yet. The code you will write will be stored in the file named Program.cs
, as shown in the following screenshot:

In Visual Studio, the Solution Explorer window on the right-hand side shows the same files as the ones in the preceding screenshot of the file system:

Tip
Some folders and files, for example, the bin folder, are hidden by default in Solution Explorer. At the top of the window is a toolbar button named Show All Files. Toggle this button to show and hide folders and files.
The C# grammar
The grammar of C# includes statements and blocks.
Statements
In English, we indicate the end of a sentence with a full stop. A sentence can be composed of multiple words and phrases. The order of words is part of the grammar. For example, in English, we say: the black cat. The adjective, black, comes before the noun, cat. French grammar has a different order; the adjective comes after the noun, "le chat noir".
C# indicates the end of a statement with a semicolon. A statement can be composed of multiple variables and expressions. In the following statement, FullName
is a variable and FirstName + LastName
is an expression:
var FullName = FirstName + LastName;
You can add comments to explain your code using a double slash //
.
The compiler ignores everything after the //
until the end of the line; for example:
var TotalPrice = Cost + Tax; // Tax is 20% of the Cost
Tip
Visual Studio will add or remove the comment (double slashes) at the start of the currently selected line(s) if you press Ctrl + K + C or Ctrl + K + U.
To write a multi-line comment, use /*
at the beginning and */
at the end of comment, as shown in the following code:
/* This is a multi-line comment. */
Blocks
In English, we indicate a paragraph with blank lines. C# indicates a block of code with curly brackets { }
. Blocks often start with a declaration to indicate what the block is defining. For example, a block can define a namespace, a class, a method, or a statement. You will learn what these are later.
In your current project, note the grammar of C# written for you by the Visual Studio template. If you are using Visual Studio 2015, the first five lines will be slightly faded out in your editor window to indicate that they aren't necessary (but leave them in for now).
In the following code block, I have added some comments and a single statement inside the Main
method:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; // ; is the end of a statement namespace Ch02_Basics { class Program { static void Main(string[] args) { // the start of a block Console.WriteLine("Hello C#"); // a statement } // the end of a block } }
The C# vocabulary
Some of the 79 predefined, reserved keywords that you will see in this chapter include using
, namespace
, class
, static
, int
, string
, double
, bool
, var
, if
, switch
, break
, while
, do
, for
, and foreach
.
Visual Studio shows C# keywords in blue to make them easier to spot. In the following screenshot, namespace
, class
, static
, void
, and string
are part of the vocabulary of C#:

There are another 25 contextual keywords that only have a special meaning in a specific context. But that still means there are only 104 actual C# keywords in the language.
English has more than 250,000 distinct words. How does C# get away with only having 104 keywords? Why is C# so difficult to learn if it has so few words?
One of the key differences between a human language and a programming language is that developers need to be able to define new "words" with new meanings.
Apart from the 104 keywords in the C# language, this book will teach you about some of the hundreds of thousands of "words" that other developers have defined. You will also learn how to define your own "words".
Tip
Programmers all over the world have to learn English because most programming languages use English words like namespace
and class
. There are programming languages that use other human languages, such as Arabic, but they are rare. This YouTube video shows a demonstration of an Arabic programming language: https://www.youtube.com/watch?v=77KAHPZUR8g.
Writing the code
Simple editors such as Notepad don't help you write correct English, as shown in the following screenshot:

Notepad won't help you write correct C# either.

Microsoft Word helps you write English by highlighting spelling mistakes with red squiggles (it should be ice cream) and grammatical errors with blue squiggles (sentences should have an upper-case first letter).

Similarly, Visual Studio helps you write C# code by highlighting spelling mistakes (the method name should be WriteLine
with an uppercase L) and grammatical errors (statements must end with a semicolon).
Visual Studio constantly watches what you type and gives you feedback by highlighting problems with colored squiggly lines under your code and showing the Error List window as you can see in the following screenshot. You can ask Visual Studio to do a complete check of your code by choosing Build-Solution or pressing F6.

Verbs are methods
In English, verbs are doing or action words. In C#, doing or action words are called methods. There are literally hundreds of thousands of methods available to C#.
In English, verbs change how they are written according to when in time the action happens. For example, Amir was jumping in the past, Beth jumps in the present, they jumped in the past, and Daz will jump in the future.
In C#, methods such as WriteLine
change how they are called or executed according to the specifics of the action. This is called overloading, which we will cover in more detail in Chapter 6, Building Your Own Types with Object-Oriented Programming. Consider the following example:
// outputs a carriage-return Console.WriteLine(); // outputs the greeting and a carriage-return Console.WriteLine("Hello Ahmed"); // outputs a formatted number and date Console.WriteLine("Temperature on {0:D} is {1}°C.", DateTime.Today, 23.4);
A different analogy is that some words are spelled the same but have different meanings depending on the context.
Nouns are types, fields, and variables
In English, nouns are names that refer to things. In C#, their equivalents are types, fields, and variables. There are tens of thousands of types available in C#.
Counting types and methods
Let's write some code to find out how many types and methods are available to C# in our simple console application.
Don't worry about how this code works. It uses a technique called reflection, which is beyond the scope of this book.
Start by adding the following statement at the top of the Program.cs
file:
using System.Reflection;
Inside the Main
method, type the following code:
// loop through the assemblies that this application references foreach (var r in Assembly.GetExecutingAssembly() .GetReferencedAssemblies()) { // load the assembly so we can read its details var a = Assembly.Load(r.FullName); // declare and set a variable to count the total number of methods int methodCount = 0; // loop through all the types in the assembly foreach (var t in a.DefinedTypes) { // add up the counts of methods methodCount += t.GetMethods().Count(); } // output the count of types and their methods Console.WriteLine($"{a.DefinedTypes.Count():N0} types with {methodCount:N0} methods in {r.Name} assembly."); }
Press Ctrl + F5 to save, compile, and run your application without the debugger attached, or click on the Debug menu and then Start Without Debugging.
You will see the following output that shows the actual number of types and methods that are available to you in the simplest application:
3,233 types with 38,529 methods in mscorlib assembly. 974 types with 9,301 methods in System.Core assembly.
Tip
The actual numbers displayed may be different depending on the version of the .NET Framework that you are using. The numbers we see here are for version 4.6.1.
Add the following four lines of code at the top of the Main
method. By declaring variables that use types in other assemblies, those assemblies are loaded with our application. This allows our code to see all the types and methods in them:
static void Main(string[] args) { System.Data.SqlClient.SqlConnection connection; System.Xml.XmlReader reader; System.Xml.Linq.XElement element; System.Net.Http.HttpClient client;
Press Ctrl + F5 and view the output in the console:
3,233 types with 38,529 methods in mscorlib assembly. 1,105 types with 14,621 methods in System.Data assembly. 1,247 types with 19,139 methods in System.Xml assembly. 91 types with 1,632 methods in System.Xml.Linq assembly. 102 types with 1,201 methods in System.Net.Http assembly. 974 types with 9,301 methods in System.Core assembly.
Now you have a better sense of why learning C# is a challenge. There are many types with many methods to learn about, and other programmers are constantly defining new ones!