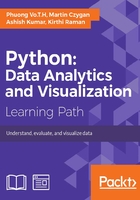
NumPy arrays
An array can be used to contain values of a data object in an experiment or simulation step, pixels of an image, or a signal recorded by a measurement device. For example, the latitude of the Eiffel Tower, Paris is 48.858598 and the longitude is 2.294495. It can be presented in a NumPy array object as p
:
>>> import numpy as np >>> p = np.array([48.858598, 2.294495]) >>> p array([48.858598, 2.294495])
This is a manual construction of an array using the np.array
function. The standard convention to import NumPy is as follows:
>>> import numpy as np
You can, of course, put from numpy import *
in your code to avoid having to write np
. However, you should be careful with this habit because of the potential code conflicts (further information on code conventions can be found in the Python Style Guide, also known as PEP8, at https://www.python.org/dev/peps/pep-0008/).
There are two requirements of a NumPy array: a fixed size at creation and a uniform, fixed data type, with a fixed size in memory. The following functions help you to get information on the p
matrix:
>>> p.ndim # getting dimension of array p 1 >>> p.shape # getting size of each array dimension (2,) >>> len(p) # getting dimension length of array p 2 >>> p.dtype # getting data type of array p dtype('float64')
Data types
There are five basic numerical types including Booleans (bool
), integers (int
), unsigned integers (uint
), floating point (float
), and complex. They indicate how many bits are needed to represent elements of an array in memory. Besides that, NumPy also has some types, such as intc
and intp
, that have different bit sizes depending on the platform.
See the following table for a listing of NumPy's supported data types:

We can easily convert or cast an array from one dtype
to another using the astype
method:
>>> a = np.array([1, 2, 3, 4]) >>> a.dtype dtype('int64') >>> float_b = a.astype(np.float64) >>> float_b.dtype dtype('float64')
Note
The astype
function will create a new array with a copy of data from an old array, even though the new dtype
is similar to the old one.
Array creation
There are various functions provided to create an array object. They are very useful for us to create and store data in a multidimensional array in different situations.
Now, in the following table we will summarize some of NumPy's common functions and their use by examples for array creation:

Indexing and slicing
As with other Python sequence types, such as lists, it is very easy to access and assign a value of each array's element:
>>> a = np.arange(7) >>> a array([0, 1, 2, 3, 4, 5, 6]) >>> a[1], a [4], a[-1] (1, 4, 6)
Note
In Python, array indices start at 0. This is in contrast to Fortran or Matlab, where indices begin at 1.
As another example, if our array is multidimensional, we need tuples of integers to index an item:
>>> a = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) >>> a[0, 2] # first row, third column 3 >>> a[0, 2] = 10 >>> a array([[1, 2, 10], [4, 5, 6], [7, 8, 9]]) >>> b = a[2] >>> b array([7, 8, 9]) >>> c = a[:2] >>> c array([[1, 2, 10], [4, 5, 6]])
We call b
and c
as array slices, which are views on the original one. It means that the data is not copied to b
or c
, and whenever we modify their values, it will be reflected in the array a
as well:
>>> b[-1] = 11 >>> a array([[1, 2, 10], [4, 5, 6], [7, 8, 11]])
Note
We use a colon (:
) character to take the entire axis when we omit the index number.
Fancy indexing
Besides indexing with slices, NumPy also supports indexing with Boolean or integer arrays (masks). This method is called fancy indexing. It creates copies, not views.
First, we take a look at an example of indexing with a Boolean mask array:
>>> a = np.array([3, 5, 1, 10]) >>> b = (a % 5 == 0) >>> b array([False, True, False, True], dtype=bool) >>> c = np.array([[0, 1], [2, 3], [4, 5], [6, 7]]) >>> c[b] array([[2, 3], [6, 7]])
The second example is an illustration of using integer masks on arrays:
>>> a = np.array([[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16]]) >>> a[[2, 1]] array([[9, 10, 11, 12], [5, 6, 7, 8]]) >>> a[[-2, -1]] # select rows from the end array([[ 9, 10, 11, 12], [13, 14, 15, 16]]) >>> a[[2, 3], [0, 1]] # take elements at (2, 0) and (3, 1) array([9, 14])
Note
The mask array must have the same length as the axis that it's indexing.
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
Numerical operations on arrays
We are getting familiar with creating and accessing ndarrays
. Now, we continue to the next step, applying some mathematical operations to array data without writing any for loops, of course, with higher performance.
Scalar operations will propagate the value to each element of the array:
>>> a = np.ones(4) >>> a * 2 array([2., 2., 2., 2.]) >>> a + 3 array([4., 4., 4., 4.])
All arithmetic operations between arrays apply the operation element wise:
>>> a = np.ones([2, 4]) >>> a * a array([[1., 1., 1., 1.], [1., 1., 1., 1.]]) >>> a + a array([[2., 2., 2., 2.], [2., 2., 2., 2.]])
Also, here are some examples of comparisons and logical operations:
>>> a = np.array([1, 2, 3, 4]) >>> b = np.array([1, 1, 5, 3]) >>> a == b array([True, False, False, False], dtype=bool) >>> np.array_equal(a, b) # array-wise comparison False >>> c = np.array([1, 0]) >>> d = np.array([1, 1]) >>> np.logical_and(c, d) # logical operations array([True, False])