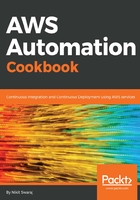
How to do it...
Now, we have both the JDK and Maven installed on our local machine. Let's create or initialize a web project in Java using Maven project template toolkit Archetype.
The Archetype plugin will create the necessary folder structure that is required by the developers to quickly start their development.
[root@awsstar ~]# mvn archetype:generate -DgroupId=com.awsstar -DartifactId=javawebapp -DarchetypeArtifactId=maven-archetype-webapp -DinteractiveMode=false
The preceding command will take some time if you are running it for the first time.
The preceding executed command will give the output in the javawebapp folder, because if you take a look at the parameter passed, -D artifactID is javawebapp.
If we go in the webapp folder and try to view the directory and file structure, then it will be as follows:

If the tree command is not present in your system, then install it by typing the command, # yum install tree. Basically, this commands shows the directory and file structure.
Maven uses a standard directory format. With the help of the output of the preceding command, we can understand following key concepts:

Apart from the source code, we need pom.xml to get built by a Maven tool. Project Object Model (POM) is a necessary part of work in Maven. It is basically an XML file. This file resides in the root directory of the project. It contains various configuration details and information about the project which will be used by Maven to build the project.
When Maven executes, it will look for the pom.xml file in the present working directory. If it finds the pom.xml file, then Maven will read the file and get all the necessary configuration mentioned in it and executes it.
Some configurations that can be specified in pom.xml are mentioned as follows:
- Developers
- Build profiles
- Project dependencies
- Mailing list
- Project version
- Plugins
- Goals
Before jumping to create a pom.xml file, we should first decide the project group (groupid), name (artifactid), and its version because these attributes will help in identifying the project. So, we can see that the command that we used to initialize in the Java Maven project contains both the project group (groupId) and name (artifactId). If we don't mention the version, then by default it takes 1.0-SNAPSHOT. The following is the snippet of our pom.xml:
[root@awsstar javawebapp]# cat pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.awsstar</groupId> -----> groupId
<artifactId>javawebapp</artifactId> -----> artifactId
<packaging>war</packaging>
<version>1.0-SNAPSHOT</version> -----> version
<name>javawebapp Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<finalName>javawebapp</finalName>
</build>
</project>
Let's try to play with the source code. By default, we have an automatic generated Hello World application in index.jsp. We need to modify and rebuild the application, and then we will try to look at the output:
[root@awsstar javawebapp]# cat src/main/webapp/index.jsp
<html>
<body>
<h2>Hello World!</h2>
</body>
</html>
Now, edit the file and put the new source code:
[root@awsstar javawebapp]# vi src/main/webapp/index.jsp
Replace the new code. Once we have the new code or feature with us, let's try to build the application:
[root@awsstar javawebapp]# mvn install
[INFO] Scanning for projects...
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] Building javawebapp Maven Webapp 1.0-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO]
[INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ javawebapp ---
[WARNING] Using platform encoding (ANSI_X3.4-1968 actually) to copy filtered resources, i.e. build is platform dependent!
[INFO] Copying 0 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.1:compile (default-compile) @ javawebapp ---
[INFO] No sources to compile
[INFO]
-------
CONTENTS SKIPPED
-------
[INFO] Webapp assembled in [18 msecs]
[INFO] Building war: /root/javawebapp/target/javawebapp.war ---> Build
[INFO] Installing /root/javawebapp/target/javawebapp.war to /root/.m2/repository/com/awsstar/javawebapp/1.0-SNAPSHOT/javawebapp-1.0-SNAPSHOT.war
[INFO] Installing /root/javawebapp/pom.xml to /root/.m2/repository/com/awsstar/javawebapp/1.0-SNAPSHOT/javawebapp-1.0-SNAPSHOT.pom
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 9.651 s
[INFO] Finished at: 2017-08-14T17:47:15+00:00
[INFO] Final Memory: 15M/295M
[INFO] ------------------------------------------------------------------------
We can see the output such as Build Success and we also get the path of the Build .war file. So the application was a web application, as mentioned in pom.xml to generate the .war file; it did the same and created a .war file in new target folder:
[root@awsstar javawebapp]# ls
pom.xml src target ------> New target folder
[root@awsstar javawebapp]# cd target/
[root@awsstar target]# ls
classes javawebapp javawebapp.war maven-archiver
Now, copy javawebapp.war created in a target folder to your webserver webapp directory and restart the webserver. Post that, try to access it:

So, basically, a Java web application initialized with Maven generally have a pom.xml for build specification and it generates a war (web archive file) in a target folder after the build happened with the mvn command.