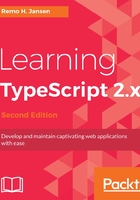
Function types
We already know that it is possible to explicitly declare the type of an element in our application by using optional type annotations:
function greetNamed(name: string): string { return `Hi! ${name}`; }
In the preceding function, we have specified the type of the parameter name (string) and its return type (string). Sometimes, we will need to not just specify the types of the function elements, but the function itself. Let's look at an example:
let greetUnnamed: (name: string) => string; greetUnnamed = function(name: string): string { return `Hi! ${name}`; };
In the preceding example, we have declared the greetUnnamed variable and its type. The greetUnnamed type is a function type that takes a string variable called name as its only parameter and returns a string after being invoked. After declaring the variable, a function, whose type must be equal to the variable type, is assigned to it.
We can also declare the greetUnnamed type and assign a function to it in the same line rather than declaring it in two separate lines as we did in the previous example:
let greetUnnamed: (name: string) => string = function(name: string): string { return `Hi! ${name}`; };
Just like in the previous example, the preceding code snippet also declares a variable, greetUnnamed, and its type. The greetUnnamed type is a function type that takes a string variable called name as its only parameter and will return a string after being invoked. We will assign a function to this variable in the same line in which it is declared. The type of the assigned function must match the type of the variable.