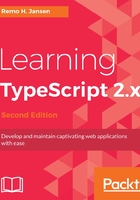
Ambient declarations
The ambient declaration allows you to create a variable in your TypeScript code that will not be translated into JavaScript at compilation time. This feature was designed to make the integration with the existing JavaScript code and the Document Object Model (DOM) and Browser Object Model (BOM) easier. Let's look at an example:
customConsole.log("A log entry!"); // error
If you try to call the member log of an object named customConsole, TypeScript will let us know that the customConsole object has not been declared:
// Cannot find name 'customConsole'
This is not a surprise. However, sometimes we want to invoke an object that has not been defined, for example, the console or window objects:
console.log("Log Entry!");
const host = window.location.hostname;
When we access the DOM or BOM objects, we don't get an error because these objects have already been declared in a special TypeScript file known as declaration files. You can use the declare operator to create an ambient declaration.
In the following code snippet, we will declare an interface that is implemented by the customConsole object. We then use the declare operator to add the customConsole object to the scope:
interface ICustomConsole {
log(arg: string) : void;
}
declare var customConsole : ICustomConsole;
We can then use the customConsole object without compilation errors:
customConsole.log("A log entry!"); // ok
TypeScript includes, by default, a file named lib.d.ts that provides interface declarations for the built-in JavaScript library as well as the DOM.
Declaration files use the file extension .d.ts and are used to increase the TypeScript compatibility with third-party libraries and runtime environments, such as Node.js or a browser.