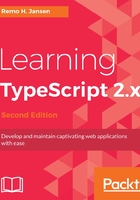
Control flow analysis
TypeScript includes a feature known as control flow analysis that is used to identify the type of a variable, based on the execution flow of a program. This feature allows TypeScript to have more precise type inference capabilities.
The following example defines a function that takes two arguments, and the type of one of them (named value) is the union type of number and array of number:
function increment(
incrementBy: number, value: number | number[]
) {
if (Array.isArray(value)) {
// value must be an array of number
return value.map(value => value + incrementBy);
} else {
// value is a number
return value + incrementBy;
}
}
increment(2, 2); // 4
increment(2, [2, 4, 6]); // [4, 6, 8]
Within the body of the function, we use an if statement to determine if the value variable is indeed an array of numbers or just a number. The type inference system will change the inferred type of the argument to match the correct type accordingly with the two paths of the if...else statement.
Control flow analysis improves the type checker's understanding of variable assignments and control flow statements, thereby greatly reducing the need for type guards.