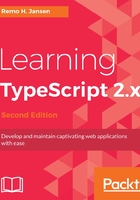
上QQ阅读APP看书,第一时间看更新
Spread operator
The spread operator can be used to initialize arrays and objects from another array or object:
let originalArr1 = [ 1, 2, 3]; let originalArr2 = [ 4, 5, 6]; let copyArr = [...originalArr1]; let mergedArr = [...originalArr1, ...originalArr2]; let newObjArr = [...originalArr1, 7, 8];
The preceding code snippet showcases the usage of the spread operator with arrays, while the following code snippet showcases its usage with object literals:
let originalObj1 = {a: 1, b: 2, c: 3}; let originalObj2 = {d: 4, e: 5, f: 6}; let copyObj = {...originalObj1}; let mergedObj = {...originalObj1, ...originalObj2}; let newObjObj = {... originalObj1, g: 7, h: 8};
The spread operator can also be used to expand to an expression into multiple arguments (in function calls), but we will skip that use case for now.