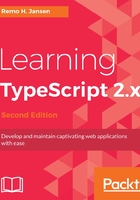
Type inference and optional static type annotations
The TypeScript language service is great at automatically detecting the type of a variable. However, there are certain cases where it is not able to automatically detect a type.
When the type inference system is not able to identify the type of a variable, it uses a type known as the any type. The any type is a value that represents all the existing types, and as a result, it is too flexible and unable to detect most errors, which is not a problem because TypeScript allows us to explicitly declare the type of a variable using what is known as optional static type annotations.
The optional static type annotations are used as constraints on program entities such as functions, variables, and properties so that compilers and development tools can offer better verification and assistance (such as IntelliSense) during software development.
Strong typing allows programmers to express their intentions in their code, both to themselves and to others in the development team.
For a variable, a type notation comes preceded by a colon after the name of a variable:
let counter; // unknown (any) type let counter = 0; // number (inferred) let counter: number; // number let counter: number = 0; // number
As you can see, we declare the type of a variable after its name; this style of type notation is based on type theory and helps to reinforce the idea of types being optional.
When no type annotations are available, TypeScript will try to guess the type of the variable by examining the assigned values. For example, in the second line, in the preceding code snippet, we can see that the variable counter has been identified as a numeric variable, because its value is a numeric value. There is a process known as type inference that can automatically detect and assign a type to a variable. The any type is used as the type of a variable when the type inference system is not able to detect its type.
Please note that the companion source code might be slightly different from the code presented during the chapters. The companion source code uses namespaces to isolate each demo from all the other demos and sometimes appends numbers to the name of the variables to prevent naming conflicts. For example, the preceding code is included in the companion source code as follows:
namespace type_inference_demo { let counter1; // unknown (any) type let counter2 = 0; // number (inferred) let counter3: number; // number let counter4: number = 0; // number }