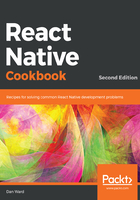
上QQ阅读APP看书,第一时间看更新
How to do it...
- Inside the App.js file, let's import our dependencies:
import React from 'react';
import { StyleSheet, View } from 'react-native';
- The App component for this app will be very simple. We just need an App class with a render function that renders our app container. We'll also add styles for filling the window and adding a white background:
export default class App extends React.Component { render() { return ( <View style={styles.container}> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', } });
- The next step for App.js will be to import and use the MainTabNavigator component, which is a new component that we'll create in step 4:
React.Component {
render() {
return (
<View style={styles.container}>
<MainTabNavigator />
</View>
);
}
}
- We'll need to create a new file for our MainTabNavigator component. Let's create a new folder in the root of the project called navigation. In this new folder, we'll create MainTabNavigator.js for our navigation component.
- In MainTabNavigator.js, we can import all of the dependencies we need for navigation. The dependencies include three screens (HomeScreen, LinksScreen, and SettingsScreen). We'll add these screens in later steps:
import React from 'react'; import { Ionicons } from '@expo/vector-icons'; import { TabNavigator, TabBarBottom } from 'react-navigation'; import HomeScreen from '../screens/HomeScreen'; import LinksScreen from '../screens/LinksScreen'; import SettingsScreen from '../screens/SettingsScreen';
- Our navigation component will use the TabNavigator method provided by react-navigation for defining the routes and navigation for our app. TabNavigator takes two parameters: a RouteConfig object to define each route and a TabNavigatorConfig object to define the options for our TabNavigator component:
export default TabNavigator({ // RouteConfig, defined in step 7. }, {
// TabNavigatorConfig, defined in steps 8 and 9. });
- First, we'll define the RouteConfig object, which will create a route map for our application. Each key in the RouteConfig object serves as the name of the route. We set the screen property for each route to the corresponding screen component we want to be displayed on that route:
export default TabNavigator({ Home: { screen: HomeScreen, }, Links: { screen: LinksScreen, }, Settings: { screen: SettingsScreen, }, }, {
// TabNavigatorConfig, defined in steps 8 and 9.
});
- TabNavigatorConfig has a little more to it. We pass the TabBarBottom component provided by react-navigation to the tabBarComponent property to declare what kind of tab bar we want to use (in this case, a tab bar designed for the bottom of the screen). tabBarPosition defines whether the bar is on the top or bottom of the screen. animationEnabled specifies whether transitions are animated, and swipeEnabled declares whether views can be changed via swiping:
export default TabNavigator({ // Route Config, defined in step 7. }, { navigationOptions: ({ navigation }) => ({
// navigationOptions, defined in step 9. }), tabBarComponent: TabBarBottom, tabBarPosition: 'bottom', animationEnabled: false, swipeEnabled: false, });
- In the navigationOptions property of the TabNavigatorConfig object, we'll define dynamic navigationOptions for each route by declaring a function that takes the navigation prop for the current route/screen. We can use this function to decide how the tab bar will behave per route/screen, since it's designed to return an object that sets navigationOptions for the appropriate screen. We'll use this pattern to define the appearance of the tabBarIcon property for each route:
navigationOptions: ({ navigation }) => ({ tabBarIcon: ({ focused }) => {
// Defined in step 10 }, }),
- The tabBarIcon property is set to a function whose parameters are the props for the current route. We'll use the focused prop to decide whether to render a colored in icon or an outlined icon, depending on the current route. We get routeName from the navigation prop via navigation.state, define icons for each of our three routes, and return the rendered icon for the appropriate route. We'll use the Ionicons component provided by Expo to create each icon and define the icon's color based on whether the icon's route is focused:
navigationOptions: ({ navigation }) => ({ tabBarIcon: ({ focused }) => { const { routeName } = navigation.state; let iconName; switch (routeName) { case 'Home': iconName = `ios-information-circle`; break; case 'Links': iconName = `ios-link`; break; case 'Settings': iconName = `ios-options`; } return ( <Ionicons name={iconName} size={28} style={{marginBottom: -3}} color={focused ? Colors.tabIconSelected :
Colors.tabIconDefault} /> ); }, }),
- The last step in setting up MainTabNavigator is to create the Colors constant used to color each icon:
const Colors = { tabIconDefault: '#ccc', tabIconSelected: '#2f95dc', }
- Our routing is now complete! All that's left now is to create the three screen components for each of the three routes we imported and defined in MainTabNavigator.js. For simplicity's sake, each of the three screens will have identical code, except for background color and identifying text.
- In the root of the project, we need to create a screens folder to house our three screens. In the new folder, we'll need to make HomeScreen.js, LinksScreen.js, and SettingsScreen.js.
- Let's start by opening the newly created HomeScreen.js and adding the necessary dependencies:
import React from 'react'; import { StyleSheet, Text, View, } from 'react-native';
- The HomeScreen component itself is quite simple, just a full color page with the word Home in the middle of the screen to show which screen we're currently on:
export default class HomeScreen extends React.Component { render() { return ( <View style={styles.container}> <Text style={styles.headline}> Home </Text> </View> ); } }
- We'll also need to add the styles for our Home screen layout:
const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', justifyContent: 'center', backgroundColor: '#608FA0', }, headline: { fontWeight: 'bold', fontSize: 30, color: 'white', } });
- All that's left now is to repeat step 14, step 15, and step 16 for the remaining two screens, along with some minor changes. LinksScreen.js should look like HomeScreen.js with the following highlighted sections updated:
import React from 'react'; import { StyleSheet, Text, View, } from 'react-native'; export default class LinksScreen extends React.Component { render() { return ( <View style={styles.container}> <Text style={styles.headline}> Links </Text> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', justifyContent: 'center', backgroundColor: '#F8759D', }, headline: { fontWeight: 'bold', fontSize: 30, color: 'white', } });
- Similarly, inside SettingsScreen.js, we can create the third screen component using the same structure as the previous two screens:
import React from 'react'; import { StyleSheet, Text, View, } from 'react-native'; export default class SettingsScreen extends React.Component { render() { return ( <View style={styles.container}> <Text style={styles.headline}> Settings </Text> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, alignItems: 'center', justifyContent: 'center', backgroundColor: '#F0642E', }, headline: { fontWeight: 'bold', fontSize: 30, color: 'white', } });
- Our application is complete! When we view our application in the simulator, it should have a tab bar along the bottom of the screen that transitions between our three routes:
