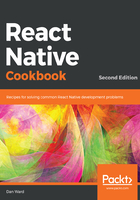
上QQ阅读APP看书,第一时间看更新
How to do it...
- We'll start by creating an images folder and adding basket.png to it. Also, create an empty file in the root of the project called sales.json.
- Inside the sales.json file, we'll define the data that we're going to display in the list. Here's some sample data:
[ { "items": 5, "address": "140 Broadway, New York, NY 11101", "total": 38, "date": "May 15, 2016" } ]
- To avoid cluttering the pages of this book, I've only defined one record, but go ahead and add more content to the array. Copying and pasting the same object multiple times will do the trick. In addition, you could change some values on the data so that each item displays unique data in the UI.
- In our App.js file, let's import the dependencies we'll need:
import React, { Component } from 'react'; import {
StyleSheet,
View,
ListView,
Image,
Text,
} from 'react-native';
import data from './sales.json';
const basketIcon = require('./images/basket.png');
- Now, we need to create the class to render the list of items. We're going to keep the sales data on the state; that way, we could insert or remove elements easily:
export default class App extends React.Component { constructor(props) { super(props); const dataSource = new ListView.DataSource({ rowHasChanged: (r1, r2) => r1 !== r2 }); this.state = { dataSource: dataSource.cloneWithRows(data), }; } renderRow(record) { // Defined in a later step } render() { // Defined in a later step } }
- In the render method, we need to define the ListView component and we'll use the renderRow method to render each item. The dataSource property defines the array of elements that we're going to render on the list:
render() {
return (
<View style={styles.mainContainer}>
<Text style={styles.title}>Sales</Text>
<ListView dataSource={this.state.dataSource} renderRow={this.renderRow} />
</View>
);
}
- Now, we can define the contents of renderRow. This method receives each object containing all of the information we need. We're going to display the data in three columns. In the first column, we'll show an icon; in the second column, we'll show the number of items for each sale and the address where this order will ship; and the third column will display the date and the total:
return ( <View style={styles.row}> <View style={styles.iconContainer}> <Image source={basketIcon} style={styles.icon} /> </View> <View style={styles.info}> <Text style={styles.items}>{record.items} Items</Text> <Text style={styles.address}>{record.address}</Text> </View> <View style={styles.total}> <Text style={styles.date}>{record.date}</Text> <Text style={styles.price}>${record.total}</Text> </View> </View> );
- Once we have the JSX defined, it's time to add the styles. First, we'll define colors, margins, paddings, and so on for the main container, title, and row container. In order to create the three columns for each row, we need to use the flexDirection: 'row' property. We'll learn more about this property in the Using flexbox to create a layout recipe later in this chapter:
const styles = StyleSheet.create({ mainContainer: { flex: 1, backgroundColor: '#fff', }, title: { backgroundColor: '#0f1b29', color: '#fff', fontSize: 18, fontWeight: 'bold', padding: 10, paddingTop: 40, textAlign: 'center', }, row: { borderColor: '#f1f1f1', borderBottomWidth: 1, flexDirection: 'row', marginLeft: 10, marginRight: 10, paddingTop: 20, paddingBottom: 20, }, });
- If we refresh the simulators, we should see something similar to the following screenshot:

- Now, inside the StyleSheet definition, let's add styles for the icon. We're going to add a yellow circle as the background and change the color of the icon to white:
iconContainer: { alignItems: 'center', backgroundColor: '#feb401', borderColor: '#feaf12', borderRadius: 25, borderWidth: 1, justifyContent: 'center', height: 50, width: 50, }, icon: { tintColor: '#fff', height: 22, width: 22, },
- After this change, we'll see a nice icon on the left side of each row, as shown in the following screenshot:

- Finally, we'll add the styles for the text. We need to set color, size, fontWeight, padding, and a few other properties:
info: { flex: 1, paddingLeft: 25, paddingRight: 25, }, items: { fontWeight: 'bold', fontSize: 16, marginBottom: 5, }, address: { color: '#ccc', fontSize: 14, }, total: { width: 80, }, date: { fontSize: 12, marginBottom: 5, }, price: { color: '#1cad61', fontSize: 25, fontWeight: 'bold', }
- The end result should look similar to the following screenshot:
